Knowledge AI Chatbot
Scaling Your AI Agent's Toolbox
Learn how to extend your AI agent with tools at scale, giving your agent the ability to perform actions and queries in your users' 3rd-party platforms
AI agents have proven to be really impressive in their ability to understand intents and perform actions with tools developers and product teams give them access to. In our last tutorial, we demonstrated how to implement function tools that interact with third-party platforms like Slack and Salesforce through Paragon workflows.
While workflows are incredibly flexible, durable, and easy to build, they may not always be the best solution for quick and simple actions. Creating a contact or sending a message are quick actions that shouldn’t require pre-built logic and can easily be handled by an AI agent.
The Manual Tooling Problem
A problem beyond workflows and third-party applications is that there are tens if not hundreds of potential tools your AI agent product may need to support - tools for web-scraping, arithmetic, querying, etc. Your engineering and product teams should spend their time on your product’s “secret sauce,” which is the underlying reasoning and routing of tool calls within your agent’s core capabilities, not manually building the functionality of each tool.
Luckily, solutions are coming onto the market that make it easy to instantly equip AI agents with access to multiple tools. In the integrations space, that solution is ActionKit, which enables developers to instantl provide hundreds of function tools to their AI agents across dozens of integrations with minimal engineering effort.

“One API to give your AI agent access to hundreds of third-party actions.”
Scaling Tool Creation for your Agent
A quick refresher on tools (AKA function tools, we’ll use both terms interchangeably). Function tools are a method for extending your agent’s capabilities by equipping it with defined functions that can be called by your agent to run a block of code. A classic example is a tool to do arithmetic. (sourced from LlamaIndex’s docs)
All function tools will follow a similar structure. The anatomy of a function tool is:
In previous iterations of Parato, we manually wrote function tools, typing up descriptions for each function and parameter to give our agent the context it needs to call a tool. This is viable and even necessary for some proprietary functions that your AI agent product may have.
There are however more agent-focused tools that makes creating function tools easier and more scalable, such as agentic.so that provides dozens of useful function tools to scrape websites, perform arithmetic, and query data sources like Twitter (X) and Wikipedia. Their node.js SDK is extremely easy to implement and scale your agent’s tools.
For building function tools with 3rd-party integrations, ActionKit is Paragon’s solution to scaling the number of function tools your AI agent has access to, making it easy to get access to hundreds of third-party actions and easily start building with them.
Tutorial Overview
This tutorial will cover how to scale the number of tools your AI agent has across integrations. We’ll go over:
How to scale the number of tools in your agent’s toolbox with ActionKit
When to use tool calls vs workflows to support specific AI use cases
How to customize the behavior of tool calls
With these topics as our north star, we’ll quickly go over what we’ve already built in our Parato tutorial series and what we’ll build in this article. (side note: If you’ve been following along our tutorial series, Parato really went through a glowup in this tutorial : ))
Quick recap
First, Parato's core functionality is a knowledge chatbot with RAG with data ingestion for integration providers like Google Drive and Notion. Paragon does the initial data pull and consumes webhook events to forward data to Parato to then index into a vector database for RAG retrieval.

Second, Parato enforces permissions by unifying third-party permissions in a single graph database to map which users have access to which documents. Similar to data ingestion, permissions are also updated with Paragon workflows to process initial permissions and stay up-to-date with permission changes.

Third, Parato can perform tool calls using third-party APIs to interact with data from your users’ external applications. In our last tutorial, we did this with workflows. This time, we’ll be using ActionKit to provide even more tools to Parato.

Let’s get started. Light, Camera, ActionKit!
Building Tools with ActionKit
ActionKit is a set of APIs that exposes 1000+ “actions” across different integrations with this type of function tool structure. We’re defining “actions” as an operation on a third-party platform - like sending a Slack message, querying a Notion page, creating a Salesforce record.
With a simple GET
request to the ActionKit API, you get access to hundreds of “actions” across dozens of integrations, each with specifically formatted JSON of the actions, descriptions, and parameters. In Parato, our GET
request to ActionKit looked like this:
The returned JSON will look like this:
This JSON format is structured exactly like the parameters needed for a function tool, meaning you can dynamically create tools with the JSON data returned from the ActionKit GET
endpoint (Note: this is just one example out of hundreds of actions. We didn’t want a massive JSON to take over the length of this article).
Performing Actions with ActionKit
While the GET
endpoint gets the descriptions and parameters needed per function tool, for the actual “function call,” we use a POST
request with the function name and parameters in the request body. Here’s an implementation with the GOOGLE_DRIVE_LIST_FILES
example from above:
The POST
request triggers the third-party action and returns the result of the Google Drive endpoint for Parato to use.
Putting it all together
ActionKit is aimed at simplifying integration actions when building AI agents. For Parato, the benefits included:
Not needing to handle third-party authentication per integration
Reading up on third-party docs to figure out parameters and their respective types per endpoint
Writing up descriptions for functions and parameters
For Parato, we wanted to expose all the possible actions for each enabled integration (that being Salesforce, Drive, Slack, Notion, Jira, and Gmail in this case but many more are available), totaling over 75 different actions. We were able to take advantage of the ActionKit benefits above, creating over 75 function tools with just a few lines of code.
For each action returned from GET
actions (the variable actions
in our example), we dynamically created a function tool from the parameters and description. The “function” that each tool will call is the POST
request to ActionKit with its name and parameters.
That’s all it took to give Parato over 75 unique tools that it can use when our agent decides a function tool is useful given its description.
Action or Workflow?
In our last tutorial, we showcased how to build function tools that triggered workflows. Does that mean ActionKit replaces workflows? It does in some cases, but workflows still have its place.
ActionKit is made up of synchronous calls perfect for simple, synchronous tasks. For example:
Sending a message in Slack or an email in Gmail is a perfect example of tasks that are simple where workflows would be overkill
Querying for a record needs to be done synchronously (immediately) for an agent to return a response back to the user in a reasonable amount of time.
Workflows, in contrast, are perfect for complex, long-running, durable, and asynchronous jobs. Use cases like:
Data ingestion, where a user could potentially trigger the ingestion of hundreds of thousands of files for indexing to a vector database is a good example of a job that’s better suited for workflows in an asynchronous nature
Syncing a users’ CRM data (for example Salesforce) to your application’s database.

In Paragon’s platform, actions are usually just a few API calls to a third-party API, whereas workflows are built on Paragon’s workflow engine allowing for parallelization of workers, resilient job queues, and more granular monitoring.
RAG Ingestion vs Query
In terms of RAG, a really interesting decision we faced when building Parato was when to query data via tool calls versus when to ingest an entire data source. We found that querying at prompt-time is better for use cases with structured data and a supported query endpoint from the integration provider.

I’ve been impressed that Parato can even write basic SQL for query actions in Salesforce.

RAG ingestion (workflows) - where we index and load textual data to a vector database asynchronously for agents to retrieve at prompt-time - is a better practice when data is unstructured and contains textual context that a simple query would not be able to search for. (If you’d like to see more context on building ingestion workflows like the Sync current files
workflow, see our first tutorial).

If you’re curious, we further researched this topic and had in depth discussions with our AI customers have about this decision. Check out his article: Beyond RAG Ingestion: A Smarter Approach to Context Retrieval.
Customizing Tool Call Behavior
Because ActionKit-enabled actions are in general a better use case for function tools than workflows, we’ll talk about how to customize the behavior of actions when an agent is ready to call a function tool.
To review, the ActionKit API benefits come from its simplicity and compatibility with function tools. When building Parato, we didn’t need to handle a lot of the difficulties that come from working with 3rd-party APIs allowing us to effortlessly integrate our AI agent with our user’s external applications.
For a more focused AI product, we probably don’t want to just give Parato every tool available, rather we’d like our users to have a good experience with the use cases and prompts they’re likely to provide Parato.
In this example, we gave Parato access to the GOOGLE_DRIVE_DELETE_FOLDER
action, but we further wanted to modify the function tool behavior to require confirmation whenever a “high-impact” action like deleting is performed. For this type of behavior, we implemented another function tool that is called before any DELETE
action and checks for confirmation.

In another example, the NOTION_SEARCH_PAGES
action returns a Notion page ID. We wanted Parato to always followup that action with the NOTION_GET_PAGE_CONTENT
action so that a user when prompting a Notion search will also immediately get back the page contents, rather than having to followup manually and ask for the contents in a subsequent prompt.

By customizing tools, we were able to be specific and create expected patterns for Parato to follow, giving a better and more predictable experience to the user.
Wrapping Up
In this tutorial, we covered a new way to scale the number of tools in your AI agent’s toolbox across integrations with ActionKit, when to use the ActionKit API vs workflows, and how to customize function tools to use actions in a way that fits your product.
We started this tutorial series with a RAG-enabled knowledge chatbot that had context from different data sources like Google Drive and Notion. We made our chatbot more secure and production-ready by processing and enforcing permissions and authorization from third-party integrations to data retrieved from the RAG process. And now, Parato is a fully functioning RAG-enabled agent that has easy access to hundreds of third-party actions via ActionKit that it can use in function tools.
We’re excited to see how Paragon can help AI companies with their use cases by supporting them with products like workflows and ActionKit. If you’d like to get your hands on Paragon’s embedded integration platform, signup for our free trial. To get access to ActionKit, schedule some time to talk to our team and book a demo!
CHAPTERS
TABLE OF CONTENTS
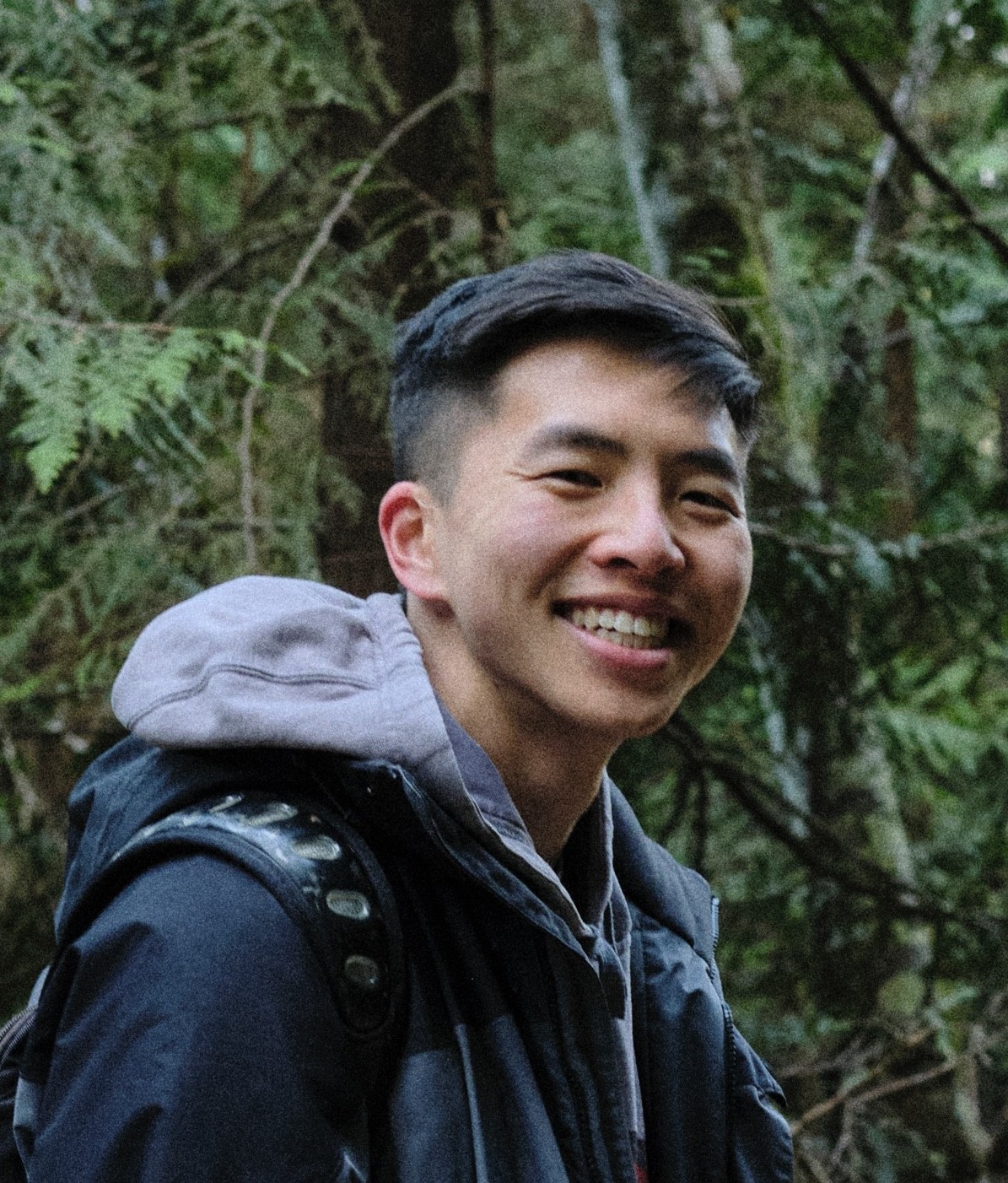
Jack Mu
,
Developer Advocate
mins to read